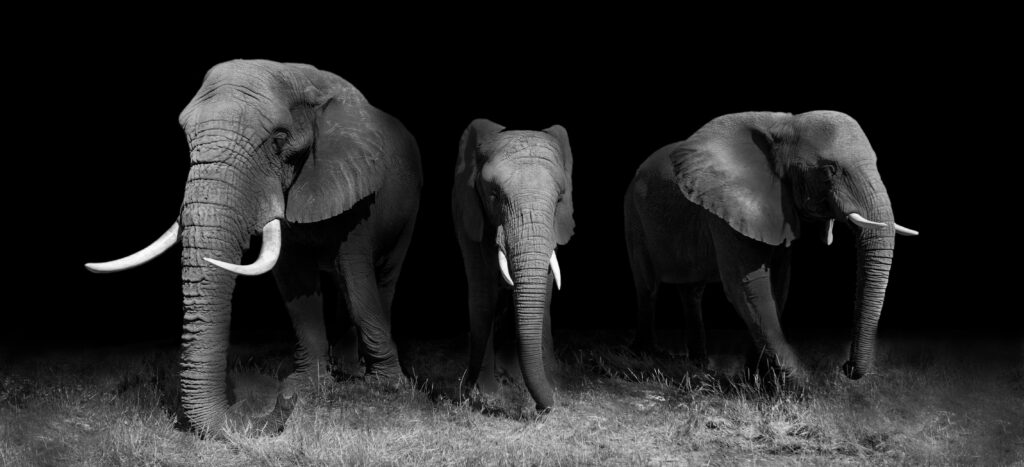
In PostgreSQL, hard parsing optimization typically takes more time when a query is submitted for the first time or when the database statistics or query planner configuration has changed significantly. This is because hard parsing involves the process of analyzing and optimizing the SQL query, which can be resource-intensive for complex queries or queries with many tables or subqueries.
When a query is submitted to PostgreSQL for the first time, it must be parsed and analyzed by the query planner, which involves evaluating the query structure, determining the best query execution plan, and optimizing the plan based on database statistics and system resources. This process can take longer for complex queries, queries with many tables or subqueries, or queries that require extensive data joins or sorting.
Similarly, hard parsing optimization can take longer when there are significant changes to the database statistics or query planner configuration, such as changes to the table schema or indexing, or changes to the PostgreSQL configuration parameters that affect query optimization. In these cases, the query planner may need to re-evaluate the query execution plan and optimize it based on the new configuration, which can be time-consuming.
To mitigate the impact of hard parsing optimization on query performance, PostgreSQL uses a query plan cache that stores previously optimized execution plans. This allows subsequent queries that are identical or similar to previously executed queries to be executed more quickly, without the need for hard parsing and optimization.
The Python script for real-time monitoring of hard parsing happening in PostgreSQL
Here is an example Python code using psycopg2 module that can be used for real-time monitoring of hard parsing happening in PostgreSQL:
import psycopg2
import time
# PostgreSQL connection details
db_name = “your_database_name”
db_user = “your_database_user”
db_password = “your_database_password”
db_host = “your_database_host”
db_port = “your_database_port”
# Connect to PostgreSQL
conn = psycopg2.connect(
dbname=db_name,
user=db_user,
password=db_password,
host=db_host,
port=db_port
)
# Open a cursor
cur = conn.cursor()
# Start monitoring for hard parsing
while True:
cur.execute(“””
SELECT pid, query, query_start
FROM pg_stat_activity
WHERE query LIKE ‘%%SELECT%%’ AND state = ‘active’
“””)
for row in cur.fetchall():
pid, query, query_start = row
print(f”Hard parsing detected. PID: {pid}, Query: {query}, Query Start Time: {query_start}”)
# Wait for 1 second before checking again
time.sleep(1)
# Close cursor and database connection
cur.close()
conn.close()
This code uses the psycopg2 module to connect to a PostgreSQL database and then runs an infinite loop that continuously monitors the pg_stat_activity view for active queries that contain the word “SELECT” in the query text. When a query is detected, the PID (process ID), query text, and start time are printed to the console.
The loop then waits for 1 second before checking again, to avoid overloading the database with too many queries.
You can customize the query to search for other types of queries or filter based on other criteria, depending on your specific use case.