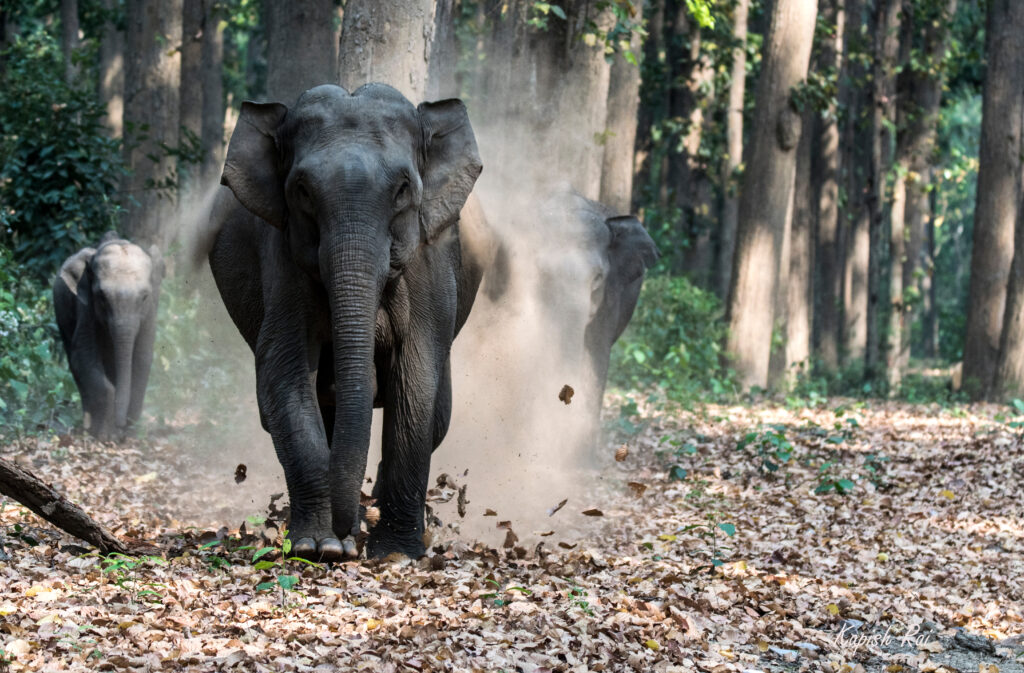
To implement bulk transactions with RESTful CRUD API using PostgreSQL and Spring Boot, you can use Spring Data JPA and Spring Data REST. Spring Data JPA provides a simple and easy-to-use interface for working with relational databases, while Spring Data REST can automatically expose your JPA repositories as RESTful resources.
Here are the general steps to implement bulk transactions with RESTful CRUD API using PostgreSQL and Spring Boot:
- Create a new Spring Boot project and configure it to use PostgreSQL.
- Define your JPA entities and repositories. For example, suppose you have a “Customer” entity with an “id” field and “name” field:
@Entity
@Table(name = “customer”)
public class Customer {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// getters and setters omitted
}
public interface CustomerRepository extends JpaRepository<Customer, Long> {
}
3. Create a new RESTful controller that exposes your JPA repositories as resources. For example, suppose you have a “CustomerController” that exposes CRUD operations for the “Customer” entity:
@RepositoryRestController
@RequestMapping(“/customers”)
public class CustomerController {
private final CustomerRepository customerRepository;
public CustomerController(CustomerRepository customerRepository) {
this.customerRepository = customerRepository;
}
@PostMapping(“/bulk”)
public ResponseEntity<List<Customer>> createCustomers(@RequestBody List<Customer> customers) {
List<Customer> savedCustomers = customerRepository.saveAll(customers);
return ResponseEntity.ok(savedCustomers);
}
}
4. Add a configuration to your application that enables bulk transactions. For example, you can add the following configuration to your “application.properties” file:
spring.jpa.properties.hibernate.jdbc.batch_size=50
spring.jpa.properties.hibernate.order_inserts=true
spring.jpa.properties.hibernate.order_updates=true
5. Test your bulk transaction by sending a POST request to the “/customers/bulk” endpoint with a JSON payload containing multiple “Customer” entities. For example, you can use the following cURL command to create two new customers:
curl -X POST \
http://localhost:8080/customers/bulk \
-H ‘Content-Type: application/json’ \
-d ‘[{“name”: “Alice”}, {“name”: “Bob”}]’
This will create two new customers in a single transaction.
By following these steps, you can implement bulk transactions with a RESTful CRUD API using PostgreSQL and Spring Boot. This approach can help improve performance and reduce the number of database round-trips required to create or update multiple entities.