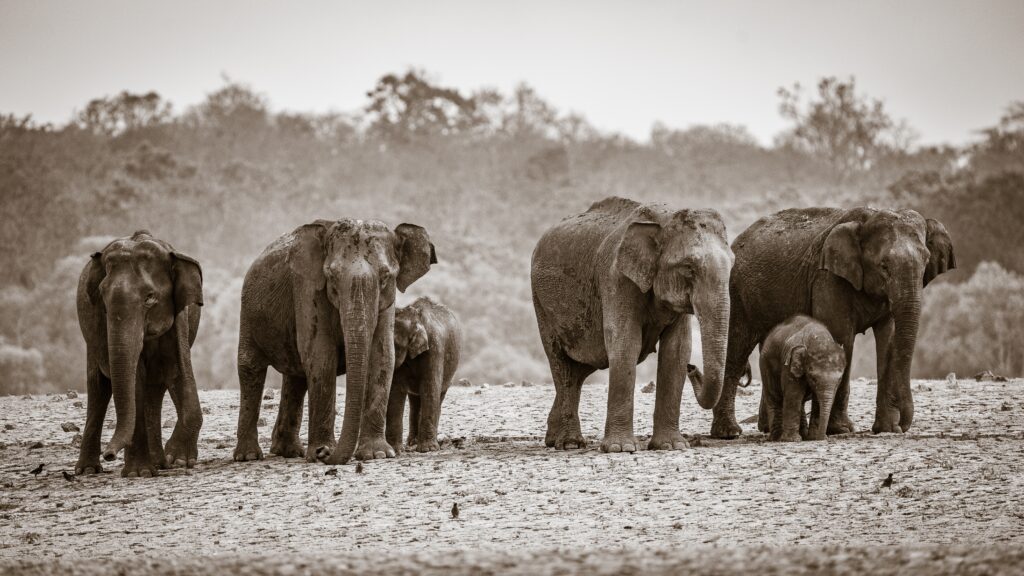
Parameterized queries and bind parameters are important concepts in PostgreSQL (and other database systems) that can help improve performance, security, and maintainability of SQL queries.
Parameterized queries are SQL queries where placeholders are used for input values instead of hard-coding values directly into the query string. Bind parameters are used to associate the placeholder with the actual value at runtime. This separation of the query logic from the input values can provide several benefits:
- Security: Using parameterized queries helps prevent SQL injection attacks by ensuring that user input is not executed as SQL code. Without parameterization, user input could be manipulated to execute malicious SQL commands.
- Performance: By using parameterized queries, the database system can cache the query execution plan and reuse it for subsequent executions with different parameter values. This can help reduce the overhead of query parsing and optimization, especially for queries with many parameters or that are executed frequently.
- Maintainability: Parameterized queries can make code more readable and easier to maintain, as the query logic is separated from the input values.
Here is an example of a parameterized query and bind parameters in PostgreSQL:
— Define the parameterized query
PREPARE my_query (text, numeric) AS
SELECT *
FROM my_table
WHERE column1 = $1 AND column2 < $2;
— Execute the query with bind parameters
EXECUTE my_query(‘some_value’, 100.0);
In this example, the PREPARE statement defines a parameterized query with two placeholders: $1 and $2. The text and numeric data types are used to specify the expected data types of the placeholders. When the query is executed with the EXECUTE statement, the actual parameter values are provided as arguments to the function call, and the database system binds these values to the corresponding placeholders in the query.
Real-life data examples of parameterized queries and bind parameters in PostgreSQL can be found in various applications that use SQL queries. For example:
- A web application that allows users to search for products by name and price. The search functionality could use a parameterized query to retrieve the matching products from the database. The user input for the name and price parameters would be bound to the placeholders in the query using bind parameters.
- A data analytics application that generates reports based on user-selected criteria. The SQL queries used to generate the reports could be parameterized queries with placeholders for the various criteria (such as date range, product type, region, etc.) The bind parameters would then be used to associate the actual values selected by the user with the placeholders in the query.
Overall, using parameterized queries and bind parameters in PostgreSQL can provide several benefits in terms of security, performance, and maintainability of SQL code. By separating the query logic from the input values, developers can write safer and more efficient code that is easier to maintain and scale.