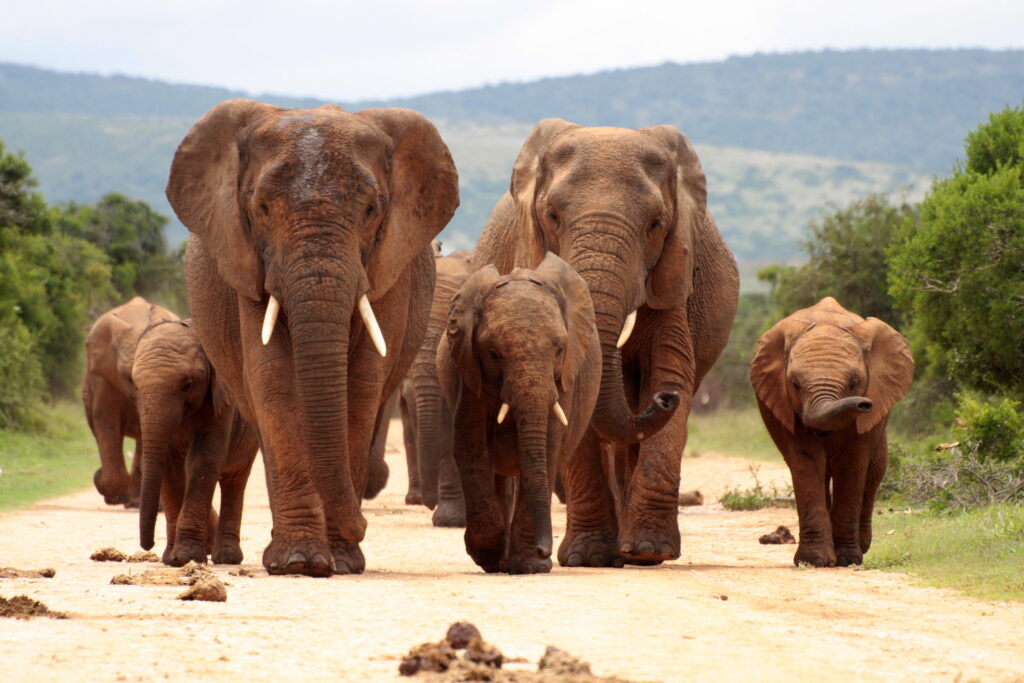
Using OR logic in outer joins can have a significant impact on PostgreSQL performance, especially when joining large tables. Here are a few factors to consider:
- Index usage: When using OR logic in the join condition, it can be more difficult for PostgreSQL to use indexes to optimize the query. In general, indexes work best when there is a single column or a small number of columns in the join condition. When using OR logic, PostgreSQL may not be able to use indexes effectively, leading to slower query performance.
- Query optimization: Queries with OR logic in the join condition can be more complex and difficult for PostgreSQL to optimize. The query planner may need to explore a larger number of possible execution plans to find the most efficient one, which can result in longer query planning times and slower query performance.
- Data distribution: When using OR logic in the join condition, the data distribution in the tables being joined can have a significant impact on query performance. If the data is distributed unevenly, with many matching records in one table and few matching records in the other, the query performance may suffer due to the need to scan a large amount of data.
- Query complexity: Queries with OR logic in the join condition can be more difficult to write and maintain. In addition, if the query involves multiple tables with complex relationships, it can be more difficult to understand and debug, which can lead to errors and slower query performance.
To mitigate the impact of OR logic on PostgreSQL performance in outer joins, you can try the following techniques:
- Use explicit type casting: When using COALESCE() to combine columns in the join condition, make sure that the data types of the columns being combined are compatible. If the data types are different, use explicit type casting to ensure that PostgreSQL can use indexes effectively.
- Optimize your data model: Try to design your data model to avoid using OR logic in outer joins whenever possible. If you need to use OR logic, consider restructuring your data or creating additional indexes to optimize the query.
- Use query optimization tools: PostgreSQL provides a variety of tools for optimizing queries, including the EXPLAIN command and query profiling tools. Use these tools to analyze your query performance and identify areas for optimization.
- Consider denormalization: In some cases, denormalizing your data by duplicating information across tables can help you avoid using OR logic in outer joins and improve query performance. However, denormalization can also make your data model more complex and difficult to maintain, so use this technique with caution.
By carefully considering the impact of OR logic on PostgreSQL performance in outer joins, and using techniques like these to optimize your queries, you can ensure that your PostgreSQL database runs efficiently and provides the performance you need.
Here are a few more real-life examples of how to incorporate OR logic when using outer joins in PostgreSQL:
- Joining on multiple columns with OR logic:
Suppose you have two tables, employees and departments, and you want to perform an outer join to find all employees and their corresponding department, where either the employee’s department ID or the department’s name matches. Here’s how you can do it using OR logic:
SELECT e.*, d.*
FROM employees e
LEFT OUTER JOIN departments d
ON e.department_id = d.id OR d.name = e.department_name;
In this example, the COALESCE() function is not needed because there are only two columns being joined. Instead, the join condition uses the OR operator to match records where either the department_id matches the id in the departments table, or the department_name matches the name in the departments table.
- Joining on multiple columns with null values:
Suppose you have two tables, orders and customers, and you want to perform an outer join to find all orders and their corresponding customer, where either the customer’s ID or the customer’s email address matches. However, some orders may not have a corresponding customer ID or email address. Here’s how you can do it using OR logic and the COALESCE() function:
SELECT o.*, c.*
FROM orders o
LEFT OUTER JOIN customers c
ON COALESCE(o.customer_id, o.customer_email) = COALESCE(c.id, c.email);
In this example, the COALESCE() function is used to combine customer_id and customer_email in the orders table, and id and email in the customers table. If either customer_id or customer_email is null, COALESCE() returns the non-null value, allowing the join condition to match records where either column matches.
By incorporating OR logic in your outer join queries using techniques like these, you can perform more complex queries and retrieve the data you need from multiple tables with greater flexibility, performance and accuracy.