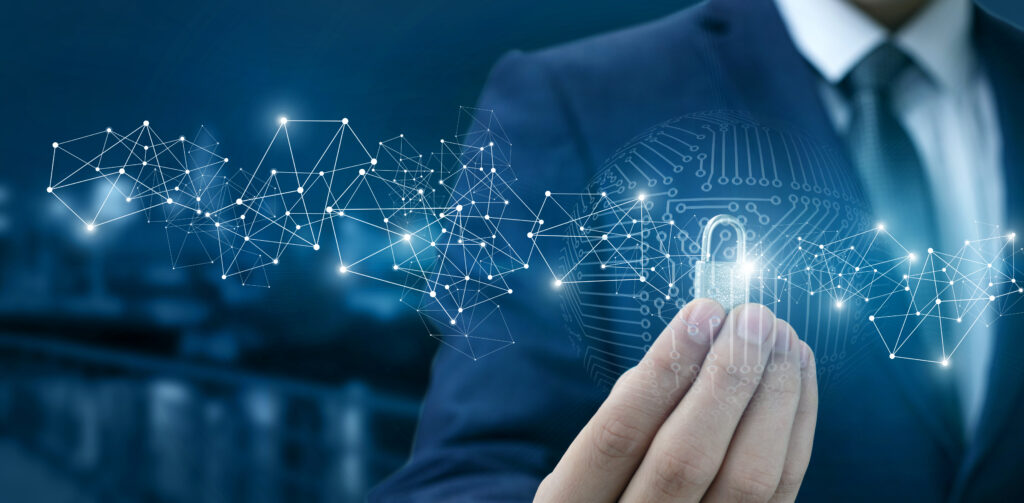
In PostgreSQL, row-level locks are implemented using a combination of lock modes and conflict resolution. When a transaction modifies a row in a table, it acquires a lock on that row to prevent other transactions from modifying it concurrently. PostgreSQL provides several lock modes, including shared locks, exclusive locks, and update locks, which allow different transactions to read and modify the data concurrently while maintaining data consistency and integrity.
Here are some practical examples of how row locks are implemented in PostgreSQL:
- Shared locks: Shared locks allow multiple transactions to read the same row concurrently, but prevent any transaction from modifying the row until all shared locks are released. For example, suppose two transactions try to read the same row in a table:
-- Transaction 1
BEGIN;
SELECT * FROM mytable WHERE id = 1;
-- Transaction 2
BEGIN;
SELECT * FROM mytable WHERE id = 1;
In this case, both transactions acquire shared locks on the row with id=1, allowing them to read the data concurrently.
- Exclusive locks: Exclusive locks prevent any other transaction from reading or modifying the row until the lock is released. For example, suppose a transaction tries to modify a row in a table:
-- Transaction 1
BEGIN;
UPDATE mytable SET status = 'complete' WHERE id = 1;
In this case, transaction 1 acquires an exclusive lock on the row with id=1, preventing any other transaction from modifying the row until the lock is released.
- Update locks: Update locks are a special type of lock that allow a transaction to read a row and later modify it without the risk of conflicting with another transaction that might also want to modify the same row. For example, suppose two transactions try to update the same row in a table:
-- Transaction 1
BEGIN;
SELECT * FROM mytable WHERE id = 1 FOR UPDATE;
-- ... perform some processing ...
UPDATE mytable SET status = 'complete' WHERE id = 1;
COMMIT;
-- Transaction 2
BEGIN;
SELECT * FROM mytable WHERE id = 1 FOR UPDATE;
-- ... perform some processing ...
UPDATE mytable SET status = 'cancelled' WHERE id = 1;
COMMIT;
In this case, both transactions acquire update locks on the row with id=1, allowing them to read and modify the data concurrently without conflicting with each other.
It is important to note that row locks can have a significant impact on the performance of the database, especially in high-concurrency environments. To optimize the performance of the database, it is important to use lock modes and conflict resolution techniques that minimize the contention for the locks and maximize the concurrency of the transactions. Additionally, it is important to monitor the database activity and identify any performance bottlenecks or lock contention issues that may arise.