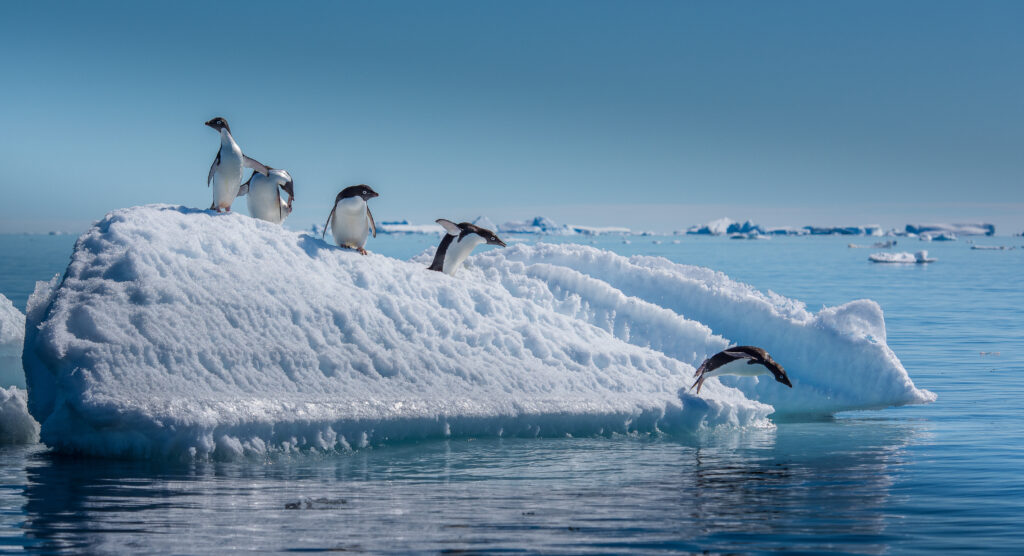
To monitor real-time Linux thread performance, we can use the psutil module in Python. The psutil module provides various system-related information, such as CPU statistics, memory usage, and process details. Specifically, we can gather information about each running process, including the number of threads it’s using.
Here’s a Python script that monitors real-time Linux thread performance using Dash for the dashboard:
# Required Libraries import dash from dash import dcc, html from dash.dependencies import Output, Input import psutil import plotly.graph_objs as go # Initialize Dash app app = dash.Dash(__name__) # App layout app.layout = html.Div([ html.H1("Real-Time Linux Thread Performance Monitoring"), dcc.Interval(id="interval-component", interval=2000, n_intervals=0), dcc.Graph(id="real-time-graph") ]) # Callback to update the graph every 2 seconds @app.callback( Output("real-time-graph", "figure"), [Input("interval-component", "n_intervals")] ) def update_graph(n): processes = [] threads = [] # Iterate over all running processes for process in psutil.process_iter(attrs=["pid", "name", "num_threads"]): info = process.info processes.append(f"{info['pid']} - {info['name']}") threads.append(info["num_threads"]) # Create a bar graph showing number of threads for each process trace = go.Bar(x=processes, y=threads) layout = go.Layout(title="Number of Threads per Process", xaxis=dict(title="Process (PID - Name)"), yaxis=dict(title="Number of Threads")) return {"data": [trace], "layout": layout} # Running the app if __name__ == "__main__": app.run_server(debug=True)
To run this script:
- Install the required modules:
pip install dash psutil plotly
Troubleshooting Linux Performance using this script:
- High Thread Count: If a process has an unusually high thread count, it could be consuming more CPU resources than expected. This might lead to reduced system performance.
- Increasing Thread Count: If the thread count for a process is consistently increasing over time, it might indicate a resource leak or an uncontrolled spawn of threads.
- Compare with Baselines: Always have a baseline to compare against. If you know the average thread count for a process during normal operation, any deviation from this baseline can indicate a problem.
- Related Metrics: While threads are an important metric, also consider other metrics like CPU usage, memory usage, and I/O operations for a comprehensive understanding.
- Resource Intensive Processes: If certain processes are using many threads, consider checking their logs or configurations to identify any anomalies.
Remember, while the thread count is a useful metric, it’s essential to consider it in conjunction with other system and application-specific metrics for a complete picture of system performance.